Write Bash Functions
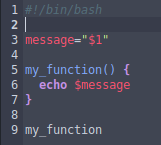
Writing bash functions is a great way to make your scripts modular. If you find you’ll be needing to repeat sets of commands or code logic, it’s a good idea to use functions to avoid redundancy in your script. Bash functions also help keep your code neat and easier to maintain.
A great way to use functions is writing a separate script with just your functions. You can then load your function script from another script as needed.
Bash functions are treated as commands. However, functions need to be defined at the top of your script. The same applies if you’re loading a script with your functions, you need to define it at the top of your script.
Writing Bash Functions
Lets take a look at some bash function examples.
This is what your typical bash function looks like:
my_function() {
echo 'This is a function.'
}
To call the function above is as follows:
my_function
Here’s what it would look like in a script:
#!/bin/bash
my_function() {
echo 'This is a function.'
}
my_function
Now lets change our function so that we can pass an argument to it.
my_function() {
echo $1
}
To use the function above, you can pass it an argument like this:
my_function 'This is a function.'
This is what it would look like in a script:
#!/bin/bash
my_function() {
echo $1
}
my_function 'This is a function.'
Now lets use a more advanced example. We will now load a function from one script to another.
We need two script files for this example. The first script we name file1.sh and the second file2.sh.
file1.sh:
#!/bin/bash
source /path/to/file2.sh 'This is a function in another script.'
file2.sh:
#!/bin/bash
message="$1"
my_function() {
echo $message
}
my_function
The first script file is calling the second script and passing it a string argument to print with the echo command within the function. The string argument we pass is held in the $message variable.
Now we would simply execute the file1.sh file and see the following output:
This is a function in another script.
That will be all for this tutorial. I hope you enjoyed writing bash functions.
Tags: tutorials, linux, command line